Learn these Python modules to be pro in admin tasks
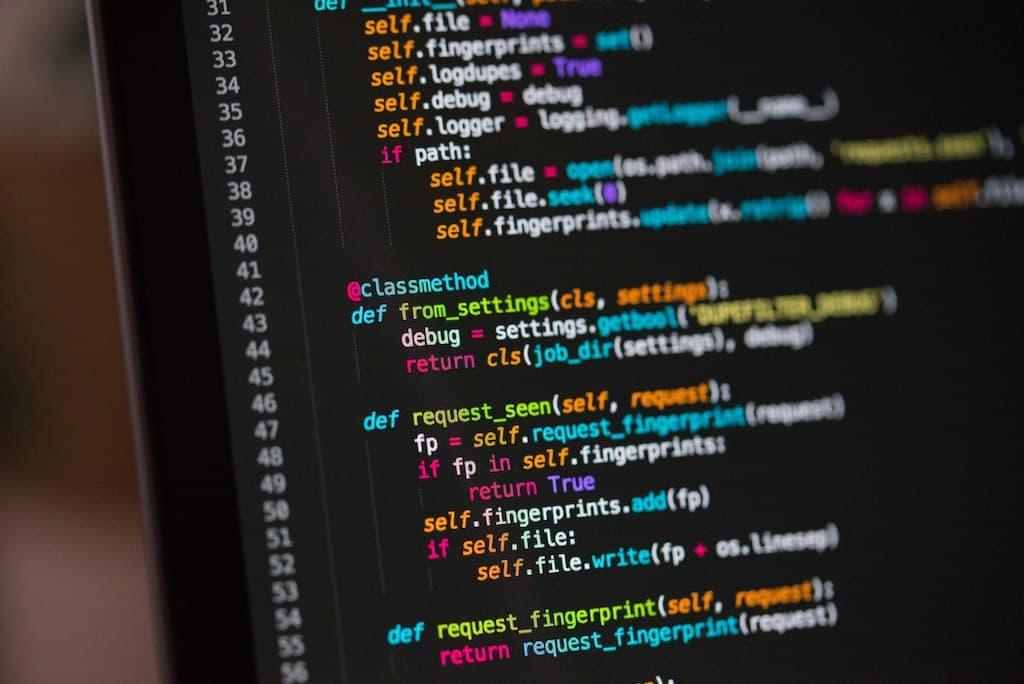
I’m not sure about you but I often hear about great achievements during Townhall mentioning about automation, every single time, without fail.
Maybe it’s because it’s easy to quantify them. A task that took 1 hour now become 5 mins. 55 mins saved every single time I need to execute that same task.
This article is written for people who wants to learn Python and are familiar with the basics of Python.
Here’s a few Python modules to improve your productivity.
win32com
win32com is a module that helps you interact with Microsoft applications. I mainly use it to write emails on Microsoft Outlook. I still recall when I change to a new role in a different company, the same piece of code just works. That's one of the reason I love open source. You write it once, you can use it forever.
Here's a function to send emails with win32com.
import win32com.client
def send_outlook_html_mail(recipient_list, subject='No Subject', body='Blank', send_or_display='Display', copies=None,
attachment=None):
"""
Send an Outlook HTML email
:param attachment: attachment path (list object in case of multiple attachments)
:param recipient_list: list of recipients' email addresses (list object)
:param subject: subject of the email
:param body: HTML body of the email
:param send_or_display: Send - send email automatically | Display - email gets created user have to click Send
:param copies: list of CCs' email addresses
:return: None
"""
if len(recipient_list) > 0 and isinstance(recipient_list, list):
outlook = win32com.client.Dispatch("Outlook.Application")
ol_msg = outlook.CreateItem(0)
str_to = ""
for recipient in recipient_list:
str_to += recipient + ";"
ol_msg.To = str_to
if copies is not None:
str_cc = ""
for cc in copies:
str_cc += cc + ";"
ol_msg.CC = str_cc
ol_msg.Subject = subject
ol_msg.HTMLBody = body
if attachment is not None:
for file in attachment:
ol_msg.Attachments.Add(Source=file)
if send_or_display.upper() == 'SEND':
ol_msg.Send()
else:
ol_msg.Display()
else:
print('Recipient email address - NOT FOUND')
glob
The glob
module in Python is used for finding all file paths that match a specific pattern.
Pattern matching
It uses patterns with wildcards like asterisk (*) and question mark (?) to match characters or any number of characters, respectively.
List of paths
The glob
function returns a list of all file paths that match the given pattern.
Applications
- Data Processing: Find specific data files (eg. all CSV Files in a directory) for analysis.
- Automation: Performing tasks on groups of files that share a naming pattern.
- Web Development: Matching files based on patterns for tasks like image processing.
Here's an example to get the latest csv file from the Downloads folder.
import glob
import os
def get_latest_file_in_download_folder(file_type):
download_folder_path = r"C:\\Users\\" + os.getlogin() + "\\Downloads\\"
download_list_of_files = glob.glob(download_folder_path + "*." + file_type)
latest_csv_file = max(download_list_of_files, key=os.path.getctime)
return latest_csv_file
latest_csv_file = get_latest_file_in_download_folder('csv')
print(latest_csv_file)
Selenium
Selenium is a powerful toolset specifically designed for automating tasks in web browsers.
It is more commonly known to use for automated web testing however it's great for automating boring web-based administrative tasks.
Here's a link to an article on selenium.
Workflow recommendation
- Download file from web based app with Selenium
- Check if file exist using
os.path.exists
- Get latest file using
os
andglob
from download folder - Email latest file to co-worker using
win32com
I came across downloads that takes a while to download so it's preferable to add some checks before reading the file.
import os
import time
def check_file_existence(file_name):
download_folder_path = r"C:\\Users\\" + os.getlogin() + "\\Downloads\\"
file_exist = os.path.exists(download_folder_path + file_name)
return file_exist
while not (check_file_existence('random_file_name.csv')):
time.sleep(0.5)
print(f'waiting for file to be downloaded')
if check_file_existence('random_file_name.csv'):
print('file downloaded')
os.path.exists
requires a file path to check if the file exist. If yes, it will return True.
The while
loop will just keep checking the file until it exist, before breaking out of the loop.
pyautogui
PyAutoGUI is a Python module designed for automating graphical user interfaces (GUIs) across different operating systems. It allows you to programmatically control your mouse and keyboard, simulating user interactions with other applications.
Here are some key features of PyAutoGUI:
- Mouse Control: Move the mouse cursor, click buttons, drag elements, etc.
- Keyboard Control: Send keystrokes, simulate typing text, use special keys.
- Image Recognition: Find images on the screen and perform actions based on their location.
- Screenshots: Capture screenshots of your entire screen or a specific region.
import pyautogui
# Move the mouse cursor to a specific location (X, Y coordinates)
pyautogui.moveTo(100, 50) # Replace 100, 50 with your desired coordinates
# Click the mouse at the current cursor position
pyautogui.click()
# Send keystrokes (e.g., press Tab key twice)
pyautogui.press(['tab', 'tab'])
# Type some text with a short pause between keystrokes
pyautogui.write("Hello, world!", interval=0.25) # Adjust interval for typing speed
# Take a screenshot of the entire screen and save it as "screenshot.png"
screenshot = pyautogui.screenshot()
screenshot.save('screenshot.png')
# Find an image on the screen (replace "image.png" with your image file)
location = pyautogui.locateOnScreen("image.png")
While selenium is good for web browser automation, we may come across desktop applications that doesn't have a DOM. PyAutoGui would be a good library to automate them.
Conclusion
All these modules are extremely powerful when combined to tackle a particular workflow.
The best way to learn is by doing.
Happy coding!